One common challenge you may come across when working with derived classes is deciding which of the two ways you should override a function in C#: using either override or new keywords.
One thing to remember is that it is necessary to declare a function `virtual` if you want to use the `override` keyword, otherwise, the override will not work, and you will get an error. The keyword `new`, however, does not require any extra refactoring, and, for these reasons, knowing how to use both override and new keywords is critical when working with derived classes.
General Setup
We will start with some basics and create two classes. One of them will be a base class and the other a derived class that inherits from the said base class. We will also create a different method in each of the classes.
Base class:
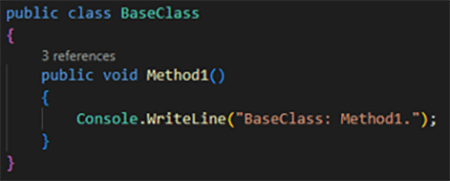
Derived class:
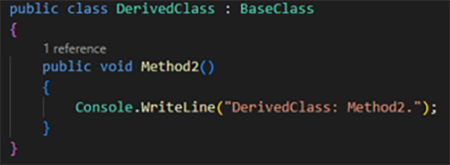
To fully demonstrate the capabilities of inheritance, we will instantiate each of the classes as separate variables and create one variable of type BaseClass that implicitly casts as such from a DerivedClass type.
Demo:
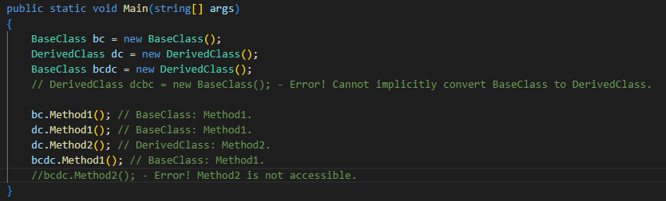
As you can see, the derived class contains both Method1 and Method2 since it has inherited all the methods from the base class.
Now, we will take a step further and make both classes implement a conflicting method. This means that both classes will implement a method that has the same signature, but different logic implementation.
Base class:
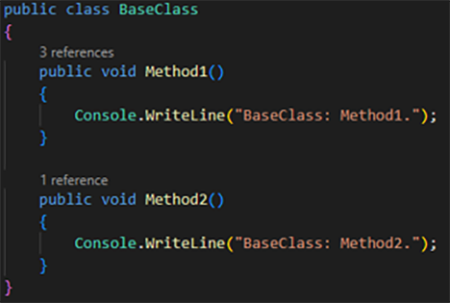
Derived class:
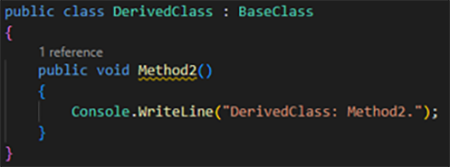
Demo:
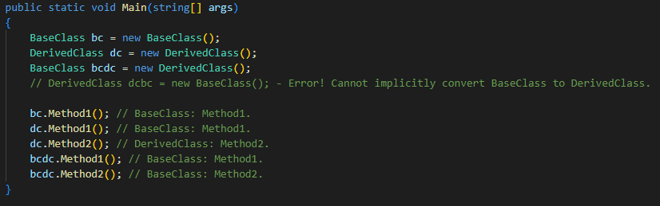
As you can see in the demonstration, you will not get an error and the code will execute. However, this is not good practice because you are making the compiler choose for you what type of an override you need, and it tells you so through a warning message. It will by default hide the method for you in the base class.
Warning message:

New
The keyword new allows you to hide a base class method. This will suppress the warning message seen in the previous example. Here is a simple way that you can use the new keyword in your code (keep in mind that you can use the keyword before or after the access keyword, in this case public):
New before public:
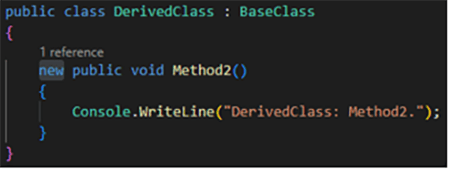
New after public:
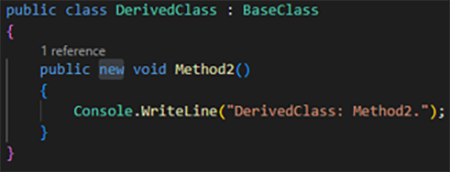
Demo:
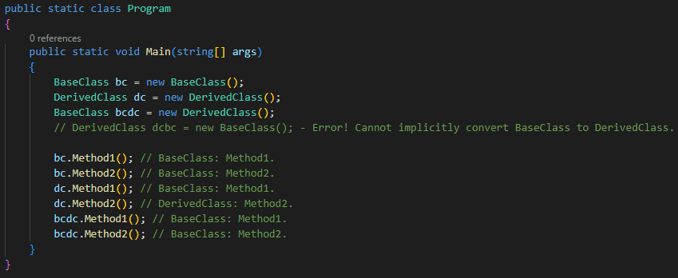
As you can see, no warning message is present and when you attempt to call Method2 in a class that was implicitly casted to BaseClass from DerivedClass you get the method implementation from the BaseClass. This simply means that when you instantiate a class as a DerivedClass you hide the original implementation of a method, so when you recast it to the Base class the original implementation resurfaces.
Override
The keyword override, on the other hand, allows you to extend a virtual method in the base class. Here is an example of how you can use the override keyword in your code (virtual and override can similarly be put before or after the access keyword):
Virtual before public:
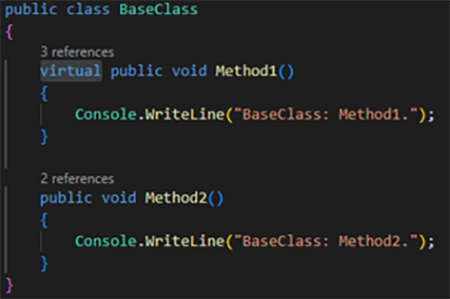
Virtual after public:
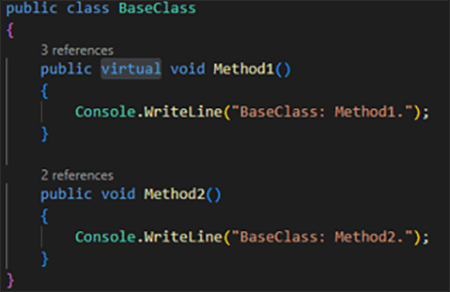
Override before public:
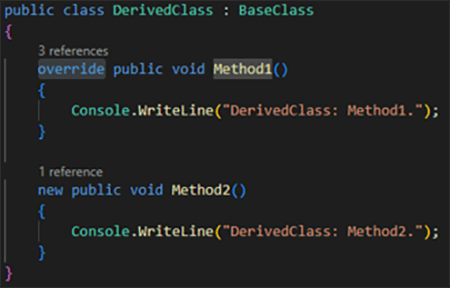
Override after public:
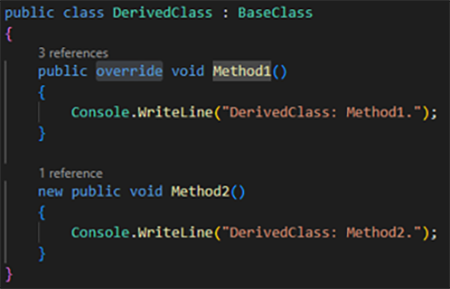
Demo:
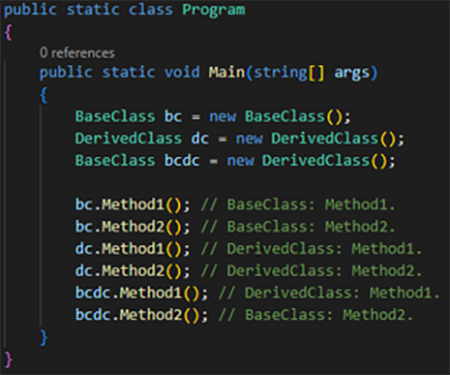
Now, when you attempt to call Method1 in the class that was implicitly casted as BaseClass from DerivedClass, the updated function implementation remains.
Note that you must implement the original function as virtual, otherwise you will see an error message preventing you from successfully compiling the code:

Example With Context
The difference between hiding and extending a method is better shown in context. Refer to the following example that completes this detailed demonstration.
Setup:
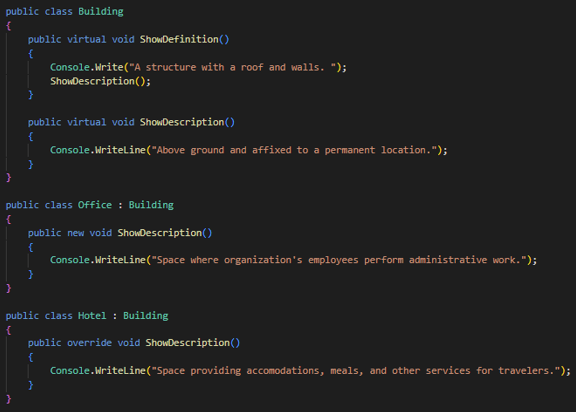
Examples:
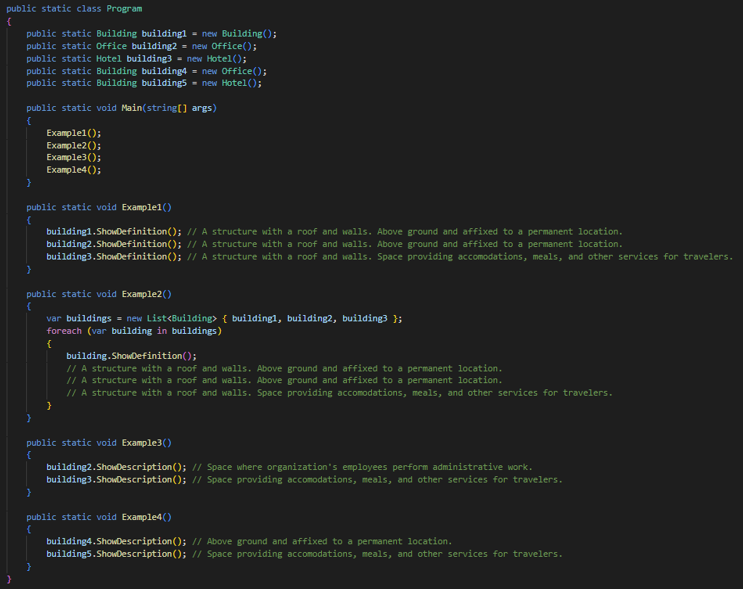
Note that in the first example building2 displays the message of the base class because ShowDefinition only has access to the base class ShowDescription method.
Learn more about DMC's C# .NET Application Development and contact us today for your next project.