From reading and writing XML files to restarting the IPC, TwinCAT PLCs offer a lot of functionality through their built-in libraries. Additionally, TwinCAT runs on a Windows environment that can offer an even wider variety of functionalities to the user. In this blog, I will be discussing how to leverage TwinCAT’s ability to send commands to the Windows command-line interface in order to expand its capabilities.
To send such commands through a TwinCAT PLC, we can use the function NT_StartProcess in the TwinCAT Utilities Library. This function simply starts a process on the Windows computer and allows you to pass in command-line parameters along with it. Therefore, with the correct syntax, we can pass in commands to the Windows command line.
Process
To start, we must make sure we import the Utilities Library. This can be done by right-clicking the references tab in the project tree and clicking "Add Library." Select the Utilities Library and press "OK."
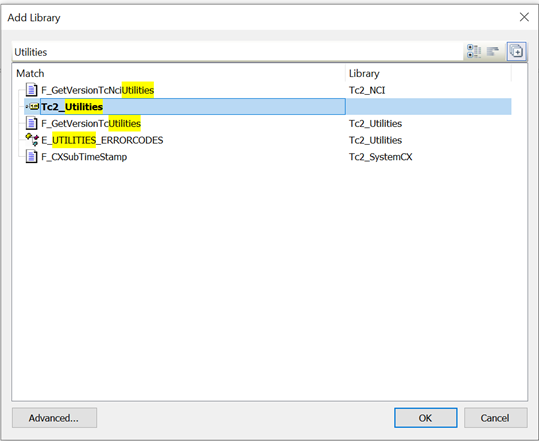
To utilize the NT_StartProcess function, you first need to locate the path to the executable. The best way to find the path is to open the command prompt and enter:
where cmd
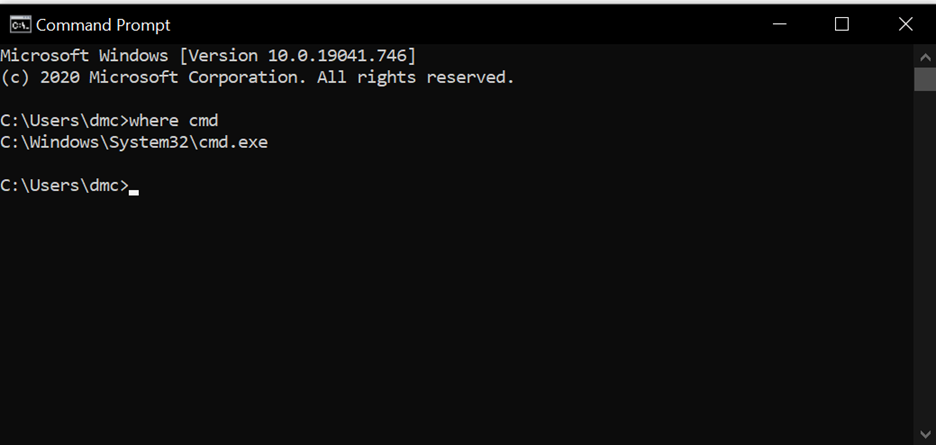
Note: The path will likely be at C:\Windows\System32\cmd.exe
Once you’ve located the path to the executable, you need to pass in the path to the command-line executable on the system as PATHSTR, and a string for the command you wish to execute as COMDLINE. In order to properly format the command string, all you have to do is prepend ‘/C ‘ to whatever command you wish to execute, making sure to include the space.
Moving Files
For this example, I’ll demonstrate how we can move a file on the Windows file system. This is something that is able to be done entirely within TwinCAT, but is much easier and quicker to do through the command-line interface. Here we are using the CONCAT function to build out our command.
// Build Command String
sCommand := '/C '; // Special command indicating command string input
sCommand := CONCAT(sCommand, 'move '); // Add move command
sCommand := CONCAT(sCommand, sTargetFilePath); // Add target file
sCommand := CONCAT(sCommand, ' '); // Required space for command
sCommand := CONCAT(sCommand, sTargetDirectory); // Add target location
Passing both strings into NT_StartProcess and triggering the START bit will cause that command to be executed.
Process(
NETID := '', // Local System
PATHSTR := 'C:\Windows\System32\cmd.exe', // Path to local cmd executable
COMNDLINE := sCommand, // Command to be executed
ERR => bError, // Error Output
ERRID => iErrorId // Error Id Output
);
You will likely not see the command prompt appear, but it may for more lengthy processes.
Keep in mind that it can be difficult to debug these command executions. If the path to the executable or the syntax of the command is incorrect, you will never see any command window pop up and likely will not receive an error. I suggest confirming the command works in an instance of the command prompt before porting it over to TwinCAT. More information on the other functionalities of the NT_StartProcess function can be found on Beckhoff’s website.
Working Example
Variable Declaration
PROGRAM MAIN
VAR
Process : NT_StartProcess;
sTargetFilePath : STRING(255);
sTargetDirectory : STRING(255);
sCommand : STRING(255);
bTrigger : BOOL := FALSE;
bError : BOOL;
iErrorId : UDINT;
END_VAR
Logic
// File Locations
sTargetFilePath := 'C:\NET-DRIVE\NewOrders\Original.xml';
sTargetDirectory := 'C:\NET-DRIVE\OldOrders';
// Build Command String
sCommand := '/C '; // Special command indicating command string input
sCommand := CONCAT(sCommand, 'move '); // Add move command
sCommand := CONCAT(sCommand, sTargetFilePath); // Add target file
sCommand := CONCAT(sCommand, ' '); // Required space for command
sCommand := CONCAT(sCommand, sTargetDirectory); // Add target location
// Output -> ‘/C move C:\NET-DRIVE\NewOrders\Original.xml 'C:\NET-DRIVE\OldOrders’
Process(
NETID := '', // Local System
PATHSTR := 'C:\Windows\System32\cmd.exe', // Path to local cmd executable
COMNDLINE := sCommand, // Comnmand to be executed
ERR => bError, // Error Output
ERRID => iErrorId // Error Id Output
);
// Trigger Command
IF bTrigger THEN
bTrigger := FALSE;
Process(START:=TRUE);
Process(START:=FALSE);
END_IF
TwinCAT natively offers a large amount of functionality to the user. The NT_StartProcess function is a great way to expand its capabilities to start programs and custom scripts from the PLC. Now, through the process described in this article, we can use the TwinCAT PLC to access the windows command prompt!
Read more about DMC’s Beckhoff and TwinCAT programming expertise and contact us to get started on your next project.