HMI Programming and SCADA Programming are essential for creating efficient and responsive automation systems. When working with Optix, leveraging NetLogic allows for seamless integration between C# code and the SCADA environment, enabling advanced control and data exchange.
NetLogic is C# code that is linked to Optix. Optix can call methods with parameters, set private C# variables, and has numerous C# Libraries integrated by default to assist with passing data between Optix and your C# code. Optix also allows you to link the monitoring of C# code to an Optix runtime instance.
Linking Variables
When creating NetLogic, you can define variables that interface with the C# code, and can be both written to and read from said C# code. You can also define variable categories to assist with organization.
- First, Variables are added onto the NetLogic object in Optix.
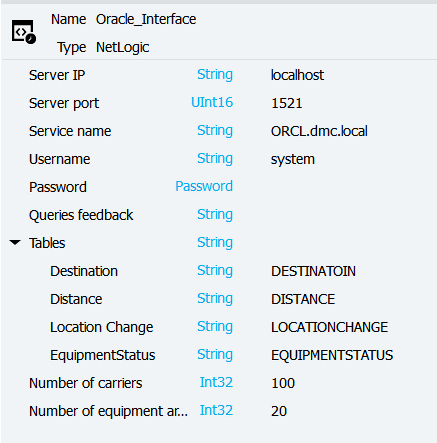
An example of variables attached to a NetLogic object
These variables can be referenced in the C# logic by utilizing the “GetVariable” method on our LogicObject.
- For any variables at the top-most level, simply call: LogicObject.GetVariable(“YourVariable”).
- For nested variables (such as variables under “Table” in the example above), define a new IUAVariable for the nested name (Tables in this case), and then reference the individual elements of that new variable.
private void ParametersSetup()
{
DB_SERVER_IP = LogicObject.GetVariable("Server IP").Value;
DB_SERVER_PORT = uint.Parse(LogicObject.GetVariable("Server port").Value);
SERVICE_NAME = LogicObject.GetVariable("Service name").Value;
DB_USERNAME = LogicObject.GetVariable("Username").Value;
DB_PASSWORD = LogicObject.GetVariable("Password").Value;
DB_QUERIES_FEEDBACK = LogicObject.GetVariable("Queries feedback");
TABLES = LogicObject.GetVariable("Tables");
TABLE_DESTINATION = TABLES.GetVariable("Destination").Value;
TABLE_DISTANCE = TABLES.GetVariable("Distance").Value;
TABLE_LOCATIONCHANGE = TABLES.GetVariable("LocationChange").Value;
TABLE_EQUIPMENTSTATUS = TABLES.GetVariable("EquipmentStatus").Value;
NUMBER_OF_DEVICES = LogicObject.GetVariable("Number of devices").Value;
NUMBER_OF_EQUIPMENT_AREAS = LogicObject.GetVariable("Number of equipment areas").Value;
CONNECTIONSTRING = $"Data Source=(DESCRIPTION=(ADDRESS_LIST=(ADDRESS=(PROTOCOL=TCP)(HOST={DB_SERVER_IP})(PORT={(int)DB_SERVER_PORT})))(CONNECT_DATA=(SERVER=DEDICATED)(SERVICE_NAME={SERVICE_NAME})));User Id={DB_USERNAME};Password={DB_PASSWORD};";
}
private IUAVariable DB_QUERIES_FEEDBACK;
private string DB_SERVER_IP;
private uint DB_SERVER_PORT;
private string SERVICE_NAME;
private string DB_USERNAME;
private string DB_PASSWORD;
public IUAVariable TABLES;
private string TABLE_DESTINATION;
private string TABLE_DISTANCE;
private string TABLE_LOCATIONCHANGE;
private string TABLE_EQUIPMENTSTATUS;
private Int32 NUMBER_OF_DEVICES;
private Int32 NUMBER_OF_EQUIPMENT_AREAS;
Calling Methods
C# Methods can be called directly from Optix when the [ExportMethod] Line is added above said method. Any parameters associated with the Method will also be exposed, allowing you to set them dynamically from Optix, using a MethodInvocation or by linking them to events.
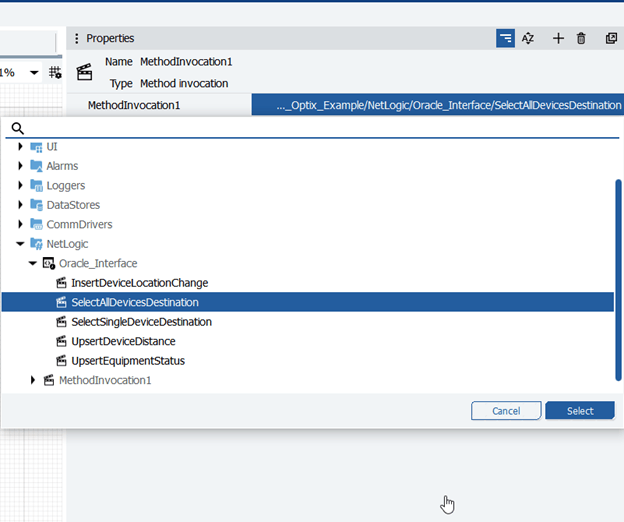
An example method invocation that shows all the [ExportMethod] methods from the Netlogic C# code
[ExportMethod]
public void SelectAllDevicesDestination()
{
…
Monitoring Code
When NetLogic is created, Optix automatically configures the codespace (if utilizing VSCode) to attach to the Optix runtime instance, allowing you to monitor your C# card while the Optix application is running.

Visual Code is attached to the Optix Runtime when monitoring
Logging and Error Handling
Optix has a C# library for logging code that should be utilized, as it will output to the console in FT Optix Studio, as well as to the log file for the Optix application.
catch (System.Exception ex)
{
Log.Error(MethodBase.GetCurrentMethod().Name, ex.Message);
}
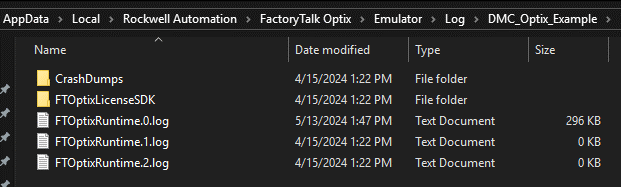
The location of Optix log files for an emulated project
Read the Other Articles in this Series
Learn more about our experience with Rockwell FactoryTalk and contact us today for your next project.